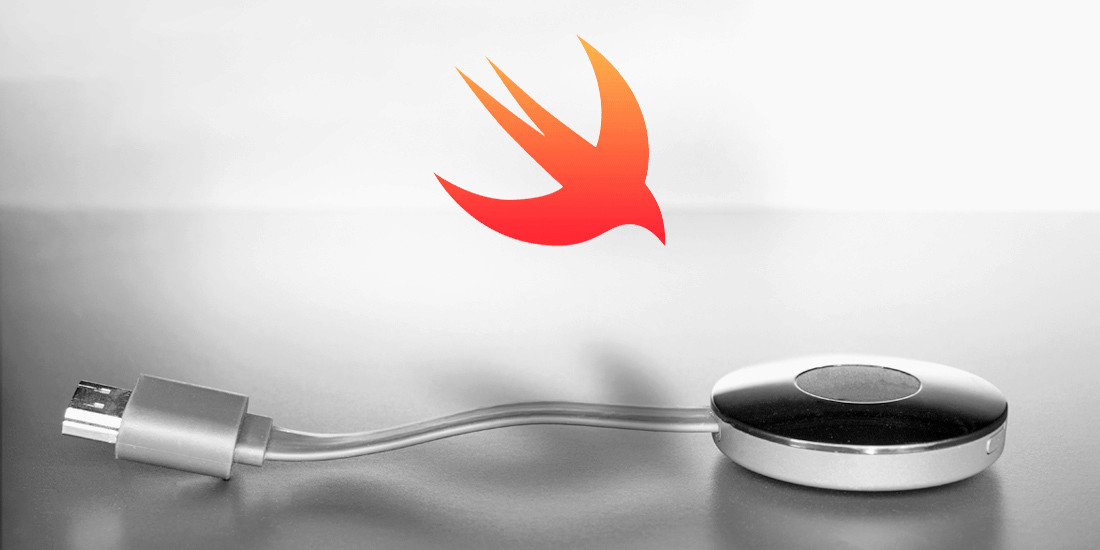
Handling Chromecast in Swift: Play and pause controls for iOS apps
Handling Google Cast support in Swift isn’t hard, but Google does not provide enough information on how to cast video or music from your app to Chromecast devices. In this tutorial, I will show you, how to handle it. Without default Google’s ViewControllers, just from your own controls.
Swift Google Chromecast support: Default Chromecast ViewControllers
- After adding the Chromecast support for your iOS app, Google offers you two options to control the casted video via dedicated ViewControllers. As we can read on their support page, we can use:
GCKUIExpandedMediaControlsViewController GCKUIMiniMediaControlsViewController
Of course, both controllers can be customized. You can not only change colors but also remove some buttons and so on. But it’s not the same as using your own play-pause controllers. You do not only pass activity to an external module but also don’t know, how it will behave. Frankly, there are also options to observe the status of a video played on Chromecast.
You can get access to
The available options are:
playing, paused, unknown, idle, buffering and loading.
So, after connecting the app with Chromecast and launching the video, you can easily check, if it is playing or paused.
GCKUICastButton
Before accessing play/pause controls, you need to set up your `GCKUICastButton`.
As you probably know, this object is used not only to launch the device picker but also for controlling the played video clips. And for detecting, if there are any Chromecast devices nearby. In some cases, the `GCKUICastButton’s` option is too much, but this can be easily resolved. If you want to disable the button’s default functions (except Chromecast detecting), simply use the flag:
`castButton.triggersDefaultCastDialog = false`
After doing this you should remember to handle the cast dialog. Otherwise, the `GCKUICastButton` won’t perform any action upon the press. You can assign the cast context action like this:
let cast = GCKCastContext()
cast.presentCastDialog()
`GCKCastContext` has two states. Firstly, it launches the View Controller with a list of available Chromecast devices. If your app is already connected with one,
This function disconnects your app from a Chromecast device:
func disconnectChromecast() {
sessionManager.endSessionAndStopCasting(true)
}
Play/pause on Chromecast
Right now we have information about the status of a casted video, and we disabled an extra player, visible via GCKCastContext. Next, we can set play and pause functions inside our app. If video isn’t loaded to Chromecast, you need to send it and launch:
func playVideoOnChromecast() {
print("enqueue item \(String(describing: mediaInfo))")
if let remoteMediaClient = sessionManager.currentCastSession?.remoteMediaClient {
let mediaQueueItemBuilder = GCKMediaQueueItemBuilder()
mediaQueueItemBuilder.mediaInformation = mediaInfo
mediaQueueItemBuilder.autoplay = true
mediaQueueItemBuilder.preloadTime = TimeInterval(UserDefaults.standard.integer(forKey: kPrefPreloadTime))
let mediaQueueItem = mediaQueueItemBuilder.build()
let queueDataBuilder = GCKMediaQueueDataBuilder(queueType: .generic)
queueDataBuilder.items = [mediaQueueItem]
queueDataBuilder.repeatMode = remoteMediaClient.mediaStatus?.queueRepeatMode ?? .off
let mediaLoadRequestDataBuilder = GCKMediaLoadRequestDataBuilder()
mediaLoadRequestDataBuilder.queueData = queueDataBuilder.build()
let request = remoteMediaClient.loadMedia(with: mediaLoadRequestDataBuilder.build())
request.delegate = self
}
}
The next function will pause the video. Of course, before the launch, you need to initialize the `GCKSessionManager`. This should be done on `viewDidLoad()`. And don’t forget to check, if the video is playing! You can do this with the `GCKMediaPlayerState` observer.
Then you can pause the video that is played on Chromecast:
func pauseVideoOnChromeast() {
sessionManager.currentCastSession?.remoteMediaClient?.pause()
}
But if the video is loaded & paused, and you want to resume, we need another solution. Otherwise (playVideoOnChromecast method), the video would be loaded again and played from the beginning.
func resumeVideoOnChromecast() {
sessionManager.currentCastSession?.remoteMediaClient?.play()
}
If we want to stop the video and load another, we should use this function:
func stopVideoOnChromeast() {
sessionManager.currentCastSession?.remoteMediaClient?.stop()
}
Handling Google Chromecast in Swift: A summary
So now we know how to use your own controls for playing, pausing, stopping and disconnecting videos sent to Chromecast devices. I didn’t show, how to launch Chromecast in your app, or how to add support for these devices. All this can be found in Google Chromecast support tutorials or source code available on Github. Hope this will help you with enabling videos from your app to Chromecast!