
Deep Links vs. Universal Links in IOS - What Are The Differences?
Learn how deep links and universal links differ from each other, spot their most important pros and cons, and, last but not least, figure out how to handle them both in iOS applications built with Swift!
Table of Content
- Deep links and universal links: Introduction to the topic
- Deep links
- Deep links in Swift apps: Implementing URL Schemes
- Implementing universal links in an iOS application
- Universal links: Adding JSON to an iOS app
- Deep and universal links in iOS: Endnote
Deep links and universal links: Introduction to the topic
Sometimes you need extra actions that can be handled from your app. For informing users about important things we have push notifications. But there are also other ways for launching applications or passing data to iOS apps. Today I’d like to show you the main differences between deep links and universal links. We’ll see how they work in Swift and how they can be handled on iOS devices.
Deep links and universal links allow launching your app from e-mail (change password link), text messages, or even websites.
They can contain extra data that can be used inside the app. Via links, you can launch a specific view that will be presented to the user. They can be very useful for automatizing some activities like login, password change, and others.
Of course, both deep links and universal links have their limitations and vulnerabilities, too.
Deep links
The main feature of deep links is that they are easy to implement.
There are no extra backend changes required or new endpoints to map. Adding deep links into your app won’t take much time.
BUT they have also cons such as asking for permission, or problems with Android, as deep links are designed mostly for iOS.
The other thing is Apple deprecated deep links in the newer iOS versions. Also, it is not recommended to use deep links due to security problems.
Deep links in Swift apps: Implementing URL Schemes
Bearing in mind the above, how to implement URL Schemes that are used for deep linking in Swift apps?
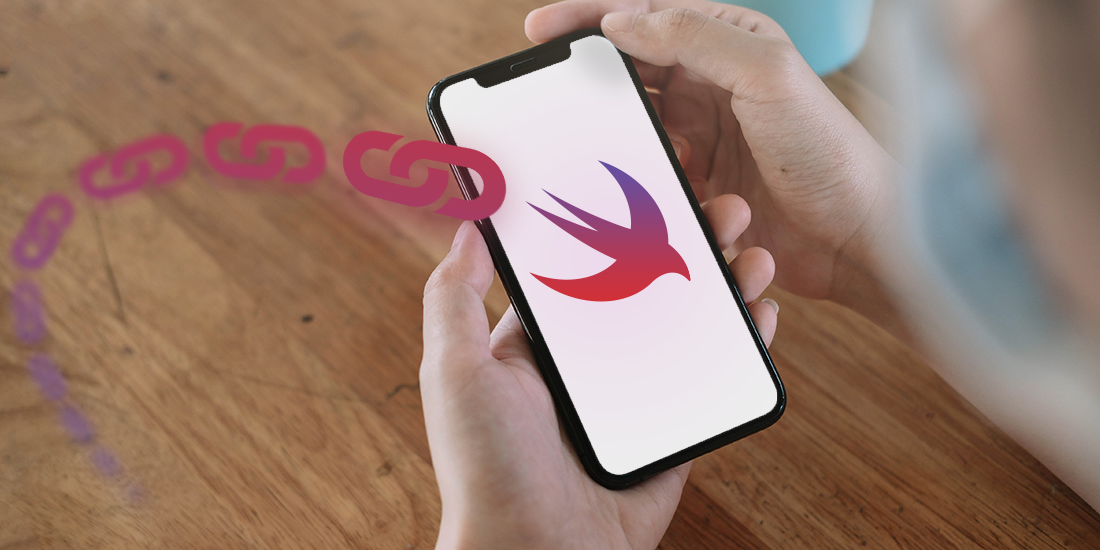
Well, firstly, we need to add an app scheme that we want to use. To do so, head over to Project Settings in Xcode, then to Info. In the section called URL Types we can add a new URL scheme. It should contain a URL scheme such as com.myAppAddress.
After adding this, we can create a simple URL link that will be recognized by our application. It must start with com.myAppAddress, and then ://myProfile.
Something like this:
com.myAppAddress://reset_password com.myAppAddress://open_custom_view_controller
This was easy. Now we need to implement these profiles. We can do it via AppDelegate or SceneDelegate. Our code will look like this:
let myUrlScheme = “com.myAppAddress”
func application(_ app: UIApplication, open url: URL,
options: [UIApplicationOpenURLOptionsKey : Any] = [:]) -> Bool {
if let scheme = url.scheme,
scheme.localizedCaseInsensitiveCompare(myUrlScheme) == .orderedSame,
let view = url.host {
var parameters: [String: String] = [:]
URLComponents(url: url, resolvingAgainstBaseURL: false)?.queryItems?.forEach {
parameters[$0.name] = $0.value
}
redirect(to: view, with: parameters)
}
return true
}
If we print the URL, we’ll get:
url.scheme = "com.myAppAddress" url.host = "open_custom_view_controller" (or whatever that will be added after "://"
URL Schemes also allow for adding parameters (look at the code above).
Thanks to this ability, you can get specific information from the link. Let’s say we want to reset a password. The link will be:
com.myAppAddress://reset_password?temp_password=1234
Then, if you print parameters, you should get ["temp_password”: 1234].
Of course, you need to have the app installed on your device to open the URL Scheme. Besides this, deep links are easy to use. But, as I said, Apple doesn’t recommend using them.
Implementing universal links in an iOS application
Now, let’s move to universal links which are currently promoted by Apple for handling cooperation between websites & apps. They’re more secure but harder to implement.
There is also a difference in the way you create links. We not only need app implementation and a specific URL link. We also need to create and keep a specific JSON file on the server-side.
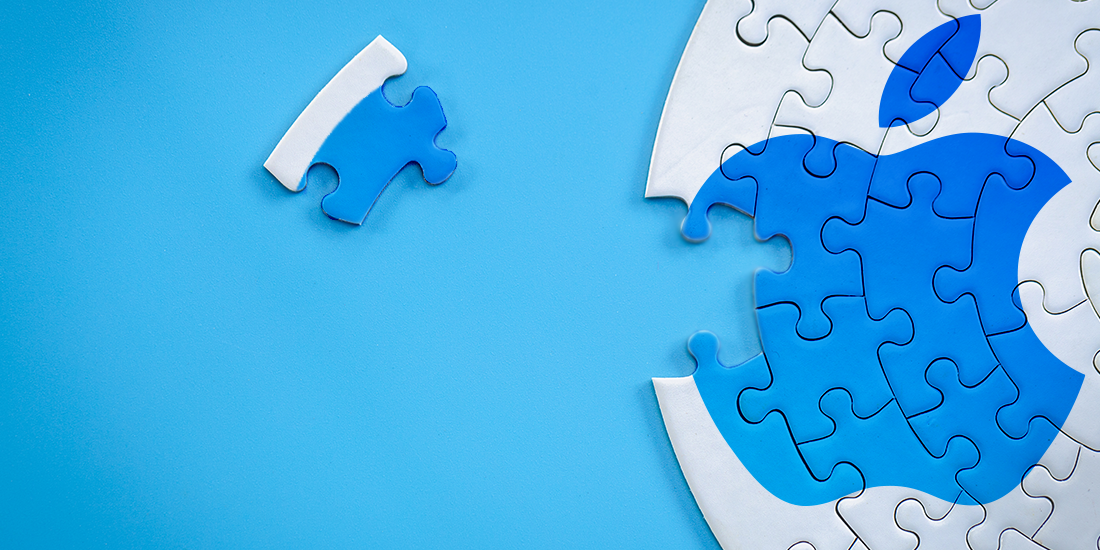
Universal links: Adding JSON to an iOS app
JSON file must be built due to Apple’s requirements. For example:
{
“applinks”: {
“apps”: [],
“details”: [
{
“appID”: “teamID.appID.specific.address”,
“paths”: [“*”],
}
]
}
}
As you can see above, in the appID field we need two elements:
- the teamID of your app,
- and an appID.
Hence, if teamID is 123456 and appID com.myApp, then the result used in the JSON file will be 123456.com.myApp.
In the paths fields, we can add paths that will be handled by an iOS app. Same as host in URL Schemes. Apple offers even more because in paths you can implement RegEx solutions for including/excluding specific strings, etc.
After adding a JSON file on the server-side let’s move on to our app.
Firstly, we need to add a new capability in Project settings. We’re talking about Associated domains. Once we enable it, we’ll need to add a URL that handles our links. For example app links:myApp.com.
When this is done, clicking the URL address in Safari should launch our app.
Now, same as with deep links, we need to handle received links. Let’s move on to AppDelegate. It should add a new function:
public func application(_ application: UIApplication,
continue userActivity: NSUserActivity,
restorationHandler: @escaping ([Any]?) -> Void) -> Bool {
if let url = userActivity.webpageURL {
var view = url.lastPathComponent
var parameters: [String: String] = [:]
URLComponents(url: url, resolvingAgainstBaseURL: false)?.queryItems?.forEach {
parameters[$0.name] = $0.value
}
redirect(to: view, with: parameters)
}
return true
}
Deep and universal links in iOS: Endnote
As you can see, similar to URL Schemes, also in this solution we have parameters that can be used in the app. But apart from this, the main difference between Universal Links and URL Schemes is that the former can be used even if the user doesn’t have the app installed. In that case, Safari will just open the link. It can lead to a default landing page or redirection to AppStore for downloading our app.
I hope this little tutorial will help you with deep & universal links in Swift apps. If you have any questions or concerns, reach out!