
How to create a Swift date handler for iOS apps?
Learn how to build a useful date handler for your iOS apps written in Swift!
Table of Content
- Date handlers and wrappers in Swift
- How to build a Swift date handler: Check your date
- Date handlers
- The Wrapper
- Summary
Date handlers and wrappers in Swift
Handling dates in a Swift project isn’t easy. You often have to change formats (Date to String, String to Date etc). Secondly, parsing Date from various types found in APIs can be a pain in the neck. Luckily, there are some tips & tricks that will help you cope with that. Wrappers and handlers are all you need.
Wrappers are useful when you want to secure your logic layer from implementation. So when in some cases you decide to abandon current solutions, you won’t have to refactor the whole code. Only the part that contains business logic. That's thanks to wrappers.
Handlers are useful, because they allow you to focus on general solutions instead of implementing logic every time. You can use singletons or extensions, or just create a separate class that will do the stuff. OK, now let’s check what we can do with date formatters in a Swift project.
How to build a Swift date handler: Check your date
If you have any problems parsing a date from an API, you can check it with NSDateFormatter.com webpage. It’s an easy skeezy date formatting website that will show you, what format string you should use for your Swift project.
Check out: The curious case of the 24-hour time format in Swift
You can simply input your date string in ISO 8601 format. Then type in the format string (e.g. MM yyy), and you’ll see the results. You can also pick a locale if you want to know what a parsed date will look like in other languages.
Apart from that, the website offers popular examples of date formats and basic date characters that are used. In general, the date formatting option can be very useful, especially when you’re not familiar with the received date format.
Date handlers
Let’s say we have a couple of types of dates that come from an API. This can be, for example, the popular JSON format:
2021-07-10T10:51:01+0000
It can be parsed with the format string yyyy-MM-dd'T'HH:mm:ssZ.
Other formats will be easy. Let’s check some examples:
- yyyy-MM-dd,
- HH:mm,
- dd.MM H:mm.
A good practice, when a date comes from API, is to parse this date as a String, then convert it to Date, and then use as a String in our views, or anywhere it is used. Probably you already do this in your projects.
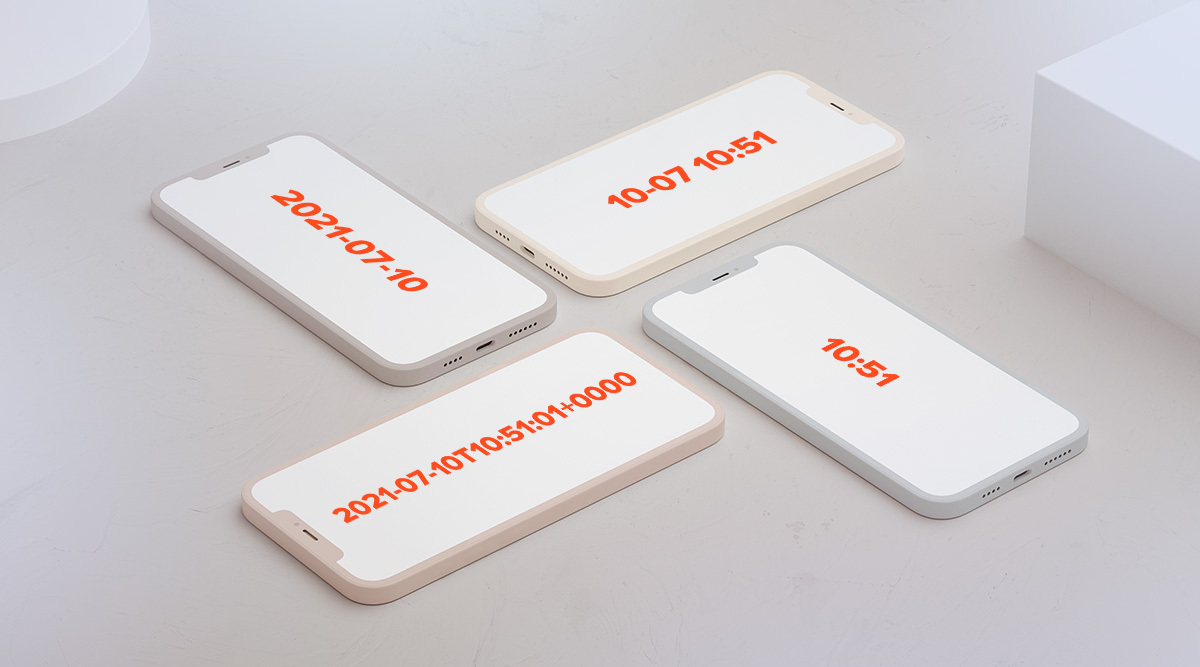
To start, we'll need an extension for DateFormatter:
extension DateFormatter {
convenience init(format: String) {
self.init()
self.dateFormat = format
}
}
This will help us implement our solutions. The next thing is to create a DateHandler structure, that will use this extension. We can do this like this:
struct DateHandler {
let formatter: DateFormatter
init(format: String) {
formatter = DateFormatter(format: format)
}
This structure may contain objects for formatting our date String. Let’s check some examples. Of course, you can add your own objects for dates.
- static let jsonDate = DateHandler(format: "yyyy-MM-dd'T'HH:mm:ssZ"),
- static let standardDate = DateHandler(format: "yyyy-MM-dd"),
- static let hourDate = DateHandler(format: "HH:mm"),
- static let fullDate = DateHandler(format: "dd.MM H:mm").
OK, the next thing. There is another hack that will help you with formatting a Date as a String. Let’s make an extension:
extension Date {
func toString(_ type: DateHandler) -> String {
type.formatter.string(from: self)
}
}
Thanks to this, you’ll only need to call the toString method on the Date object.
let someDate = Date()
let stringFromDate = someDate.toString(.fullDate)
Nice, huh? And it isn’t my final form! We can do similar stuff with a String object - i.e. change it to Date with single line. Our extension for String will look like this one:
extension String {
func toDate(_ type: DateHandler) -> Date? {
type.formatter.date(from: self)
}
}
Implementing this extension is very easy, same as with Date:
let someString = "21:37"
let dateFromString = someString.toDate(.hourDate)
As we can see, this solution lets you implement the Date changer with a single line. It works in both directions: from Date to String, and from String to Date. You can use it in views or in classes for mapping JSON from API.
The Wrapper
At last, we should implement a wrapper. This will be easy. Just create yet another struct that will return our DateHandler. Let’s call it DateWrapper. DateWrapper has only one task: to return DateHandler which contains methods transforming Date to String and String to Date. So let’s add this solution:
struct DateWrapper {
let handler: DateHandler
init(format: String) {
handler = DateHandler(format: format)
}
}
Then, in extensions, we need to change the type from DateHandler to DateWrapper. Thanks to this, we’ll have a wrapper return DateHandler right now and other solutions when needed.
Swift date handler: Wrapping up
I presented you the methods that I’m using for changing Date into other objects (mostly Strings). If you find it interesting, try to implement in your projects! See you in the next post on our site!