
Custom UIAlertController Swift: How To Make It?
In this short tutorial I will show you how to make a custom UIAlertController
which can be used on many View Controllers or even Storyboards. The main advantage of this solution is that your alert controller can be used many times and modified as you wish. OK, let’s start with the basics!
A default UIAlertController
is very simple and, of course, not easy to modify. In fact, it offers only a few options. So if you want your own, custom alert view, you should design a UIView
with buttons, labels etc. That custom alert view can be used on your UIViewController
. But how to use the same custom alert view for more than one view?
UIContainerView
can be the solution. For more details check Apple documentation. In this tutorial, I'm going to show only one simple solution of UIContainerView
, so if you use more than one Storyboard in your project, please create a new one. If you don’t - you can skip this and jump to the next point.
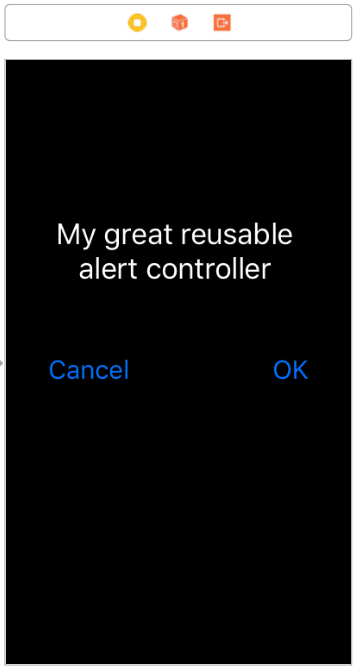
Creating a new UIViewController
Now, we need to create a new UIViewController
. Let’s call it AlertViewController
. Now we can add buttons and labels etc. to our view - i.e. everything that is needed for your alert view. A good option is to resize the View Controller
to have a better idea how it will present itself on our main view. Besides you should add proper constraints to UIView
containing all needed objects.
When this is done, all constraints & outlets are set, let’s go to a place where we are going to use our newly created alert view. Now we can use UIContainerView
to easily place the new controller. Just add UIContainerView
to the controller and add constraints. This kind of view is automatically added with external view. You can find it outside your controller. Now, delete this view. Next, with the CTRL key, drag the line from the UIContainerView
beyond the controller - up till the AlertViewController
. Out of possible segue types, pick Embed
which is called on viewDidLoad
.
The whole action should look like this:
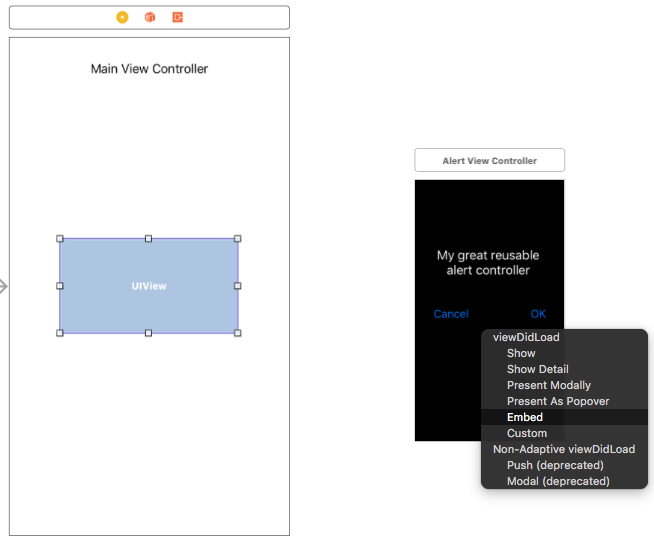
This was easy. Now our AlertViewController
is linked with the UIContainerView inside the other UIViewController
and should be launched automatically on func viewDidLoad
.
As I mentioned before, this kind of alert can be used on many controllers. In fact, you can make a separated Storyboard just for AlertViewController
. And then, by using the Storyboard
reference object, link it with other controllers. Piece of cake.
Last but not least. How to pass data from UIViewController
and AlertViewController
placed in UIContainerView
? Simply use protocols. You can also call the Embed
segue programmatically on viewDidLoad
function.
override func viewDidLoad() {
super.viewDidLoad()
performSegue(withIdentifier: "showAlertViewController", sender: self)
}
Of course, previously you need to create a segue from the main View Controller
to AlertViewController
on the Storyboard and call it for example "showAlertViewController"
.
In case you are hungry for more knowledge, let me show you how to make a reusable Activity Indicator with Swift! Have fun!
Photo by Jean Gerber on Unsplash