
Starting with Rails - how to set up a development environment
Take your very first steps with Ruby! This tutorial will show you how to set up an environment for future development in Ruby on Rails.
This (very) basic tutorial is dedicated to everyone who, without prior experience, wants to 'taste' coding and get started with Ruby on Rails. I assume that if the subject matter of this post interested you enough to read these words, you're already familiar with the basics and know what terms like Ruby, Rails, dev environment or terminal are. Still, you might not have had the opportunity to do anything about it in practice. If so, let me take you for a walk and step-by-step show you that coding is a very satisfying brain-teaser. We're going to work with the current stable versions, in our case it's Ruby -v 2.5.1, Rails -v 5.2.
How to set up the ruby on rails development environment?
Rbenv installation guide
So, let's start setting up ruby on rails development environment. I use OSX, so my configuration will be dedicated to this operating system, however, if you'd like to go through this tutorial on Linux you'll find out that the installation is very much alike. I skip installation on systems supported by Windows deliberately. If you’re thinking about learning how to code - switching to Linux or Unix-based operating system is strongly recommended.
The first thing we have to do is to install the Ruby version management tool (also called version control tool or package manager). It will allow us to manage all (not only stable) Ruby versions and switch them accordingly in different projects, if that's necessary. Among several (major and minor) options here are two key players to choose from: rbenv and RVM. Let's skip digging into details when choosing which one to use, the Internet is full of comparisons, you can easily google the differences. In this tutorial I'm going to use rbenv
script as I find it more user-friendly.
To install rbenv
follow the instructions from this link: https://github.com/rbenv/rbenv#installation
Just to be sure we're on the same page: on OSX it is recommended to install rbenv
with additional package manager called Homebrew, or just brew https://brew.sh/. To install Homebrew just paste the following in your terminal:
$ /usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
(Tip: when you see a command that is prefaced with the $
sign, it means that it should be placed in a command line of your terminal)
This command should take you through the installation, follow the instructions displayed by your console.
Now, when the installation is completed, let the brew install rbenv
:
$ brew install rbenv
Type a command-line rbenv
in your terminal to see if rbenv
is properly installed. Terminal should return the actual rbenv
version and some useful commands.
Our next step is to integrate rbenv
with our shell by calling rbenv init
command:
$ rbenv init
Now you need to add a line to your shell's configuration file, so that it loads rbenv
automatically every time you open the console. Typically, when you enter the terminal, it loads commands listed in a specific file in your home directory - depending on the system and shell you use, it may be named .bashrc, .zshrc or .bash_profile. To check this, execute the following command:
$ ls -a ~
and look for one of these filenames.
Then, execute:
$ echo 'eval "$(rbenv init -)"' >> ~/.bash_profile
Use ~/.bash_profile if you didn’t find .bashrc or .zshrc (even if .bash_profile does not exist either). Otherwise, replace it with one of the files you found.
Afterwards, the next time you open the shell, it will be aware of rbenv. Now, open the new shell.
Ruby installation using rbenv
Mind the gap: rbenv
will not install Ruby on your machine automatically. You have to download it by yourself with providing the version you need. The most recommended version is, surprise surprise, the most current (and stable) version. If you’d like to check your possibilities, use the command
$ rbenv install --list
to see all available versions, then choose the best (once again, current and stable) one. In my case, the 2.5.1 is the number, so I’m typing:
$ rbenv install 2.5.1
and the installation starts.
Summing up: to install Ruby is to provide the version you need, the rest of this process happens automatically so you don’t have to configure anything on your own or dig deep into compilations.
Having installed the newest version of Ruby, all you have to do is to ‘force’ your system to use this version globally as a default.
$ rbenv global 2.5.1
To make sure it worked type:
$ rbenv versions
The terminal should turn back the following info which should assure you that everything is OK, as so far.
- 2.5.0 (set by ~/ruby/.ruby-version)
Gem setup
Now it’s time to say a few words about gems. ‘Gem’ is a library with a particular functionality (user authentication, geolocation or search engine) you can (and surely will) use in the Ruby environment.
Here’s the thing: some gems are integrated with Ruby itself (json for example). Some additional gems are automatically bundled with the Ruby itself during the instalation (rake for example, here you can find the list of rest of them: https://github.com/ruby/ruby/blob/v251/gems/bundled_gems). The rest of gems you have to download separately (including Rails, yes, Rails is a gem too!).
You can manage those libraries (gems) in two ways:
1) globally for the current (activated) Ruby version via RubyGems (link: https://rubygems.org/) by executing the gem install
command (which installs any gem you want, anytime you want),
2) locally (per project) via the Bundler (http://bundler.io) library - if you start working with a new Rails project, all needed gems will be specified in a special file called Gemfile.
You also need a gem dependency manager called Bundler. Bundler cleans the mess by tracking and installing the exact gems and versions you will need in your Rails projects.
Install a Bundler gem:
$ gem install bundler
Now let’s install Rails. It will be installed with some additional gems (about 40 for Rails 5.2.1) and will also make Rails commands available in the shell including the one you’re going to use to create the application.
$ gem install rails
Congratulations, from now on your system recognizes the ‘Rails’ command as something actionable.
To check if everything works fine, you can use command gem list
which will return a list of all installed gems.
Now the tools are ready, the environment is properly set up but nothing functions (yet). The most important step is to create a new project, which results in generating a whole directory structure.
Type:
$ rails new my_first_rails_app
Now, let’s stop for a while and take a short look at the simplicity of Rails. The above words, rails + new + name, written in your shell do the whole magic for you and give you your Rails app, out of the box. Several complicated processes are hidden behind this ‘rails new’ command. Rails creates the whole directory structure with all the system folders (in my case ~/my-first-rails-app), Gemfile directory, and runs bundle install
.
Now, enter this directory:
$ cd my_first_rails_app
Good job, you have reached a new milestone and created your first Rails project!
Finally, time for the last step: turning on the Rails server. To do it, call it in your terminal:
$ bin/rails server
(you can also use the shorter version $ bin/rails s)
Now, your local server (http://localhost:3000) should welcome you with a message:
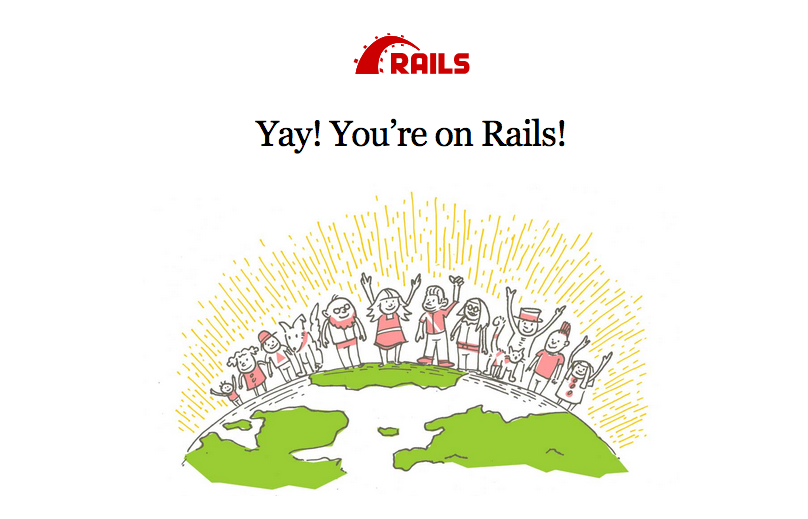
Congratulations! From now on, you’re ready to create your first application with Ruby on Rails.
How to continue with programming?
Last year I participated as an attendee in the Rails Girls Poznan event. Rails Girls is a global movement that encourages women to get into tech world by possessing the experience in coding by learning Ruby on Rails. The above post was created thanks to the knowledge I gained during the Poznan's workshops. For those of you who'd like to contiunue learning and are eager to find out more about RoR development: the Internet is full of free resources and online courses. Besides them, don't forget about written resources and make sure to have Best Books to learn Ruby on Rails on your bookshelf.
Update: Rails 6.0 is finally here!
After over two years and thousands of commits, Rails 6.0 has finally been released. The new version of the framework comes with features such as Action Mailbox, Action Text, parallel tests, multiple database support* and **Zeitwerk. How can they bring value to your Rails app? Read the article in which we discuss them in detail and comment on how can they impact the future of Ruby on Rails.
photo from: gratisography.com and author's resources